Introduction
Astro is a web framework well-suited for content-driven websites. Its Islands Architecture allows you to combine static pages with dynamic elements, striking a balance between performance and interactivity. Beyond that, you can leverage SSR, middleware, and endpoints to build applications that run on the server side, too.
Recent updates have especially emphasized features for building dynamic sites, hinting at Astro’s evolution into a robust, full-stack framework. For a more detailed introduction to Astro, check out the official Why Astro? docs.
In this post, I’ll summarize what I see as the most significant happenings in the Astro ecosystem in 2024. I’ll also touch on what might be coming in 2025, as far as I’m aware.
If you’re interested, I’ve also published a 2023 edition (in Japanese) of this article. Feel free to check it out for a look back at Astro’s progress in 2023.
Project health indicators
Release Versions
As of the start of 2024, Astro was at v4.0.8, and by the time of writing this article near the end of the year, v5.1.1 has been released. Including beta versions, there were a total of 169 releases in 2024. That’s almost on par with the number of releases in 2023, which was 175.
In 2023, the major version jumped from 1 to 4, giving the impression of a rapid pace of feature additions and improvements. In 2024, the major version only went from 4 to 5, and the pace of version bumps seems to have eased somewhat.
For those who are curious about the details of each release, you can check the full list of Astro releases on GitHub.
GitHub Stars
According to GitHub Star History, Astro’s GitHub stars were around 37,000 in early 2024 but increased to around 48,000 by the end of the year. Although that’s a bit slower than 2023’s increase of 15,000, it’s still a jump of over 10,000 for the year. It’s fair to say Astro’s star count is still on a healthy upward trend.
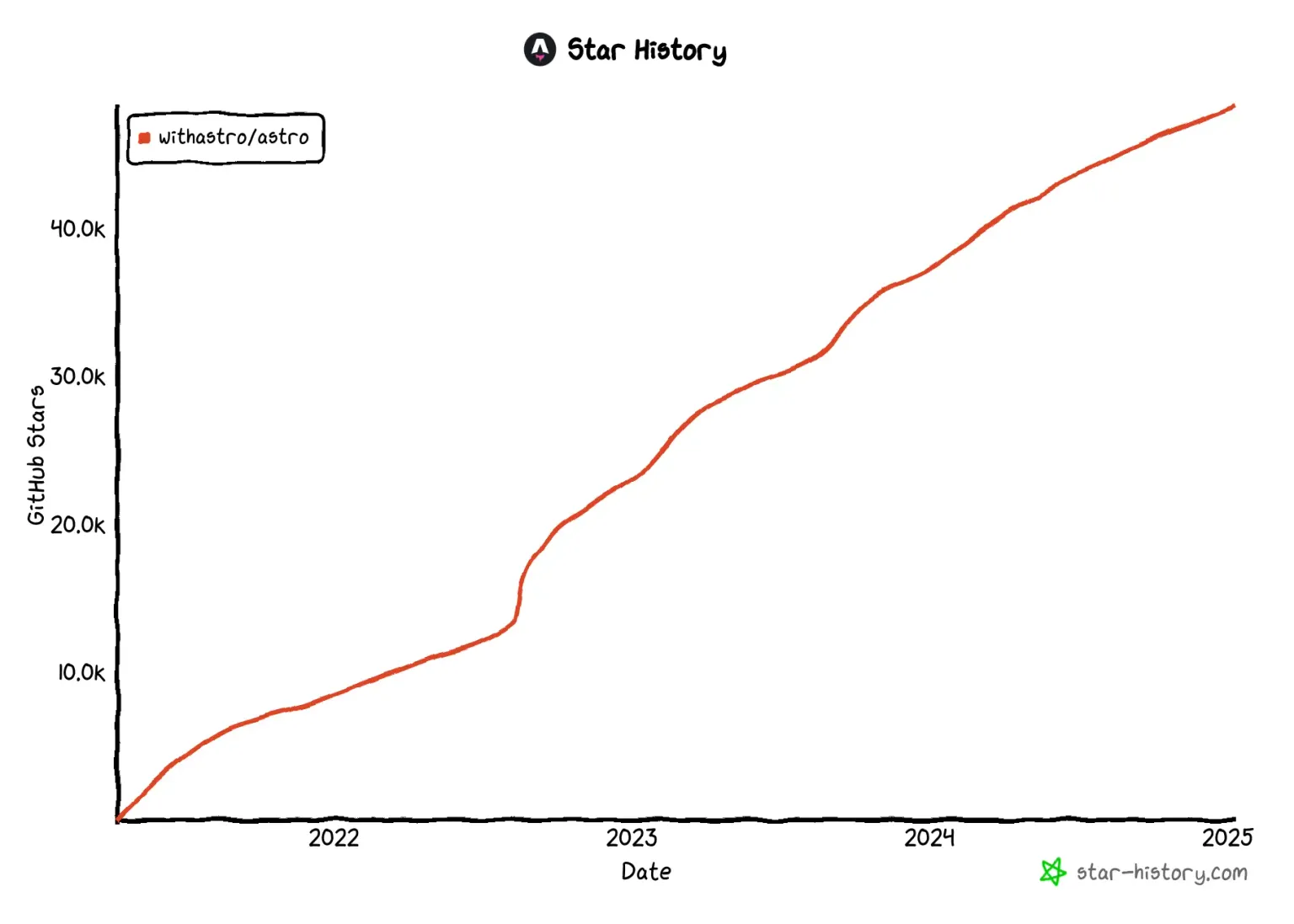
NPM Downloads
Based on npm trends, weekly downloads of Astro were 185,902 at the start of 2024, climbing to 364,201 as of December 15. That’s approximately double, so Astro is clearly growing at a steady pace here as well.
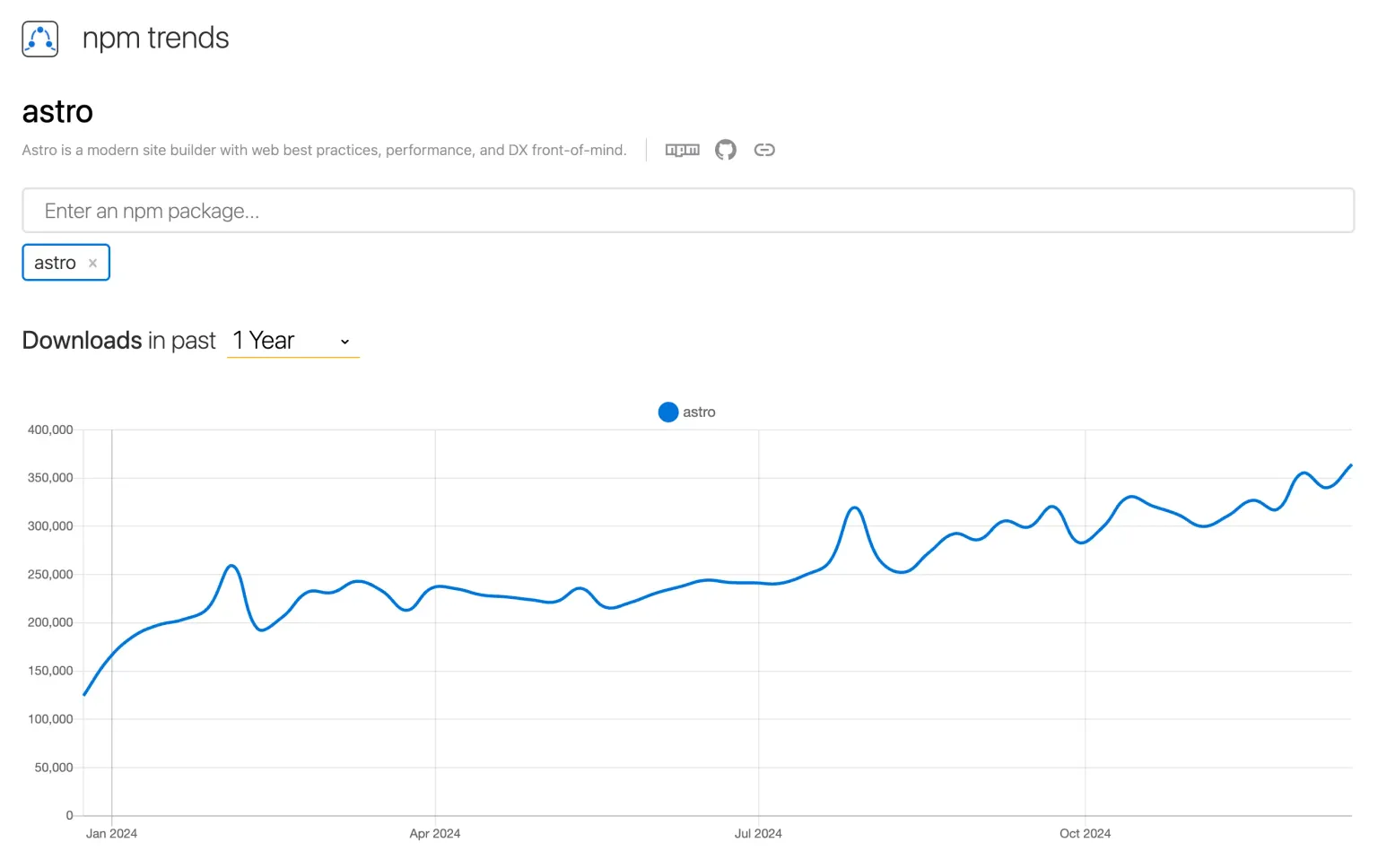
State of JavaScript
In the Meta Frameworks category of State of JavaScript 2024 published in December, Astro ranked #1 in:
- Interest (the proportion of positive sentiment among respondents having heard about an item)
- Retention (the proportion of positive sentiment among respondents having used an item)
- Positivity (the proportion of positive sentiment among respondents who expressed a sentiment)
On top of that, its Usage (the proportion of respondents having used an item) rose from 4th to 2nd this year, trailing only Next.js:
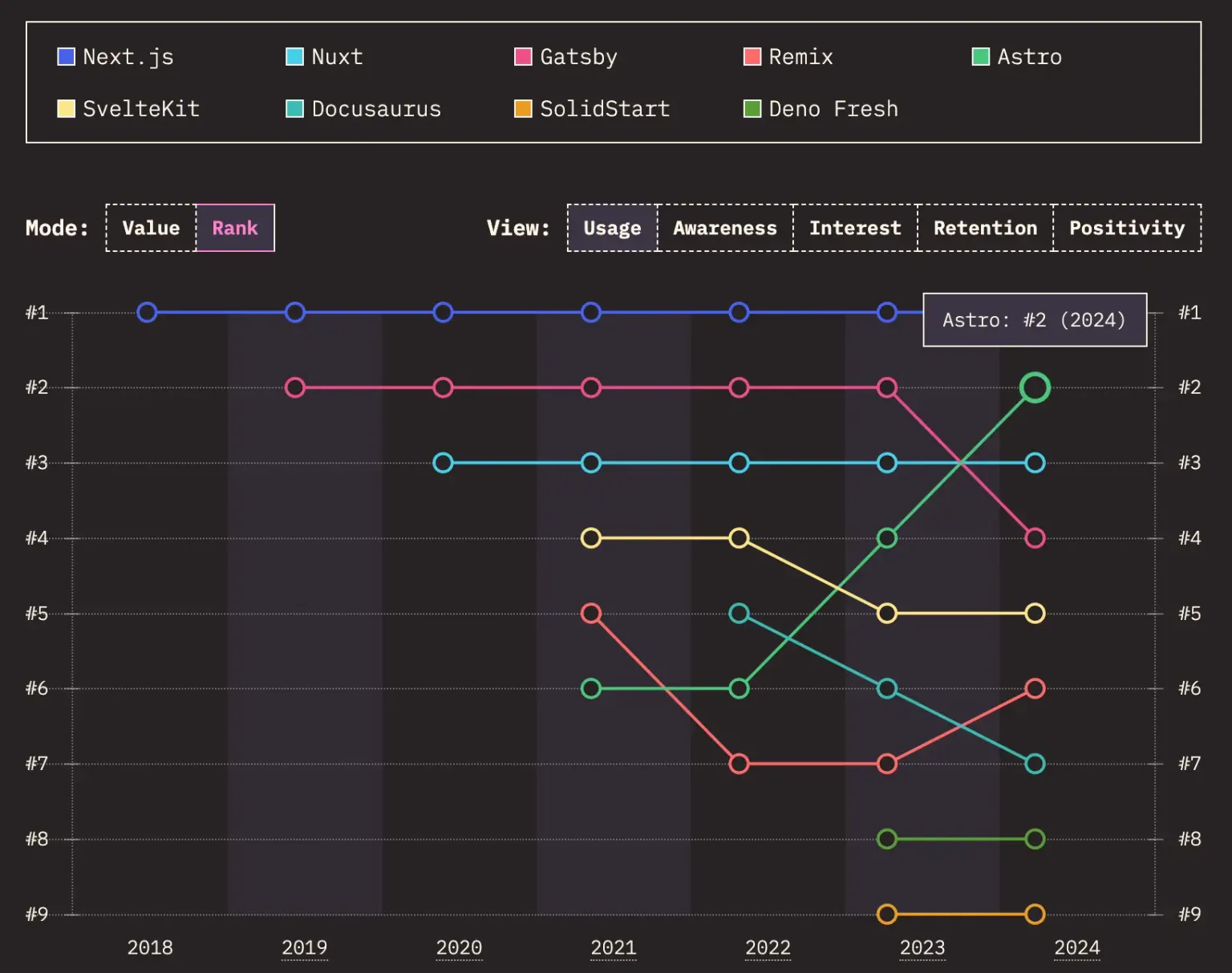
Astro also climbed to 5th place in Awareness (the proportion of respondents having heard about or used an item).
Overall, every metric was either “an improvement over last year” or “still holding the #1 spot”. Based on the State of JavaScript survey, 2024 can be seen as a pivotal year of progress for Astro.
New and Updated Features
Content Layer
Content Collections were introduced in Astro 2.0 as a way for type-safe handling of local content written in Markdown, etc. In Astro 5.0, the new Content Layer API was added to expand how content is defined, making it even more flexible and powerful for various use cases.
Previously, Astro’s Content Collections could only handle content like Markdown or MDX files located locally in the reserved src/content
directory:
- src/content/ - blog/ - post-1.md - post-2.md
Your content configuration schema could then validate the frontmatter of each Markdown file, provide editor autocompletion when dealing with content data retrieved by the getCollection
function, and so on.
With the new Content Layer API introduced in v5.0, you can keep the validation and autocompletion benefits while extending definitions for your content. For example, you can now “load” external API data as a collection with the new loader
property:
import { defineCollection, z } from "astro:content";
const countries = defineCollection({ loader: () => fetch("https://api.example.com/countries").then(res => res.json()), schema: z.object({ /* schema definition here */ }),});
export const collections = { countries };
Under this new Content Layer, type
(which you previously provided to defineCollection
) is now considered legacy, and the loader
is where you specify how data should be loaded, making it possible to handle any kind of data source, not just local files.
In v5, you get two built-in loaders for your local content, glob()
and file()
, making it easy to do things like:
const blog = defineCollection({ loader: glob({ pattern: "**/*.md", base: "./src/data/blog" }), schema: z.object({ /* schema definition here */ }),});
This blog collection behaves similar to the old local file-based approach. Meanwhile, because loader
can be any function, you can also pull data from external APIs or databases, or even generate content on the fly.
In this way, the new Content Layer generalizes how content is handled in Astro and also allows you to freely define and distribute custom data loaders, significantly expanding Astro’s capabilities around content. The Content Loader API for building custom loaders also comes with handy features like the meta
key-value store for storing metadata about your content and the rendered
field for storing rendered output.
With the newly refreshed Content Collections backed by the Content Layer API, go ahead and create your own loader—if it turns out well, publish it as an npm package and share it with the Astro community in the “Content Loaders” category of the integrations library! For instance, Storyblok, Cloudinary, and Hygraph have already released their own loaders, so you can fetch data from these services without having to write your own loader from scratch.
Check out the official docs to learn more about Content Collections.
Astro Actions
Astro already offered endpoints for handling HTTP requests on the server side. But in v4.15, Astro Actions were introduced to make it easier to call backend services less boilerplate-y and more type-safe. Some of the benefits of Astro Actions include:
- Automatic input validation using Zod
- Automatic generation of type-safe functions that invoke your actions
- Standardized error handling through the
ActionError
type and theisInputError
function
Here’s an example of how you might define an Action in src/actions/index.ts
:
import { defineAction } from "astro:actions";import { z } from "astro:schema";
export const server = { // Define an action that takes a name and returns a greeting getGreeting: defineAction({ // The schema for the input sent to this action input: z.object({ name: z.string(), }), // The server-side handler for this action handler: async (input) => { return `Hello, ${input.name}!`; // input is validated and can be accessed type-safely } })}
Note that you don’t see code for checking Content-Type
, performing input validation, etc. Astro Actions handle that boilerplate so that you can focus on the core business logic.
Then, on the client side:
------
<button>Get greeting</button>
<script>// Import the `actions` object that contains the `getGreeting` actionimport { actions } from "astro:actions";
const button = document.querySelector("button");button?.addEventListener("click", async () => { // Call the `getGreeting` action const { data, error } = await actions.getGreeting({ name: "Actions" }); // Show the result in an alert if there's no error if (!error) alert(data);})</script>
By importing actions
from astro:actions
, you get a function to call your getGreeting
action. Thanks to the schema you provided, you can call getGreeting
type-safely. And of course, you can hover over the action in your editor to see the input and return types or cmd+click
to jump to its definition.
Moreover, you can use the isInputError
function to check if error
is a validation error, or throw ActionError
within your action handler to set specific HTTP status codes, etc.
Given its name, Astro Actions are influenced by React’s Server Actions (Functions). Both attempt to solve similar problems, but Astro Actions seems to go a step further with out-of-the-box validation and structured error handling. You might not need extensive server-side communication in an Astro project, but you can slot Actions in as needed—for example, adding a Like feature on a blog post.
Check out the official docs to learn more about Astro Actions.
Server Islands
A new rendering approach called Server Islands has been introduced in Astro 5.0. Astro’s Islands Architecture has always allowed selective hydration of certain portions of a page, but now Server Islands extends that concept to let you render parts of your page on the server. Below is the image shared in the initial announcement for Server Islands:
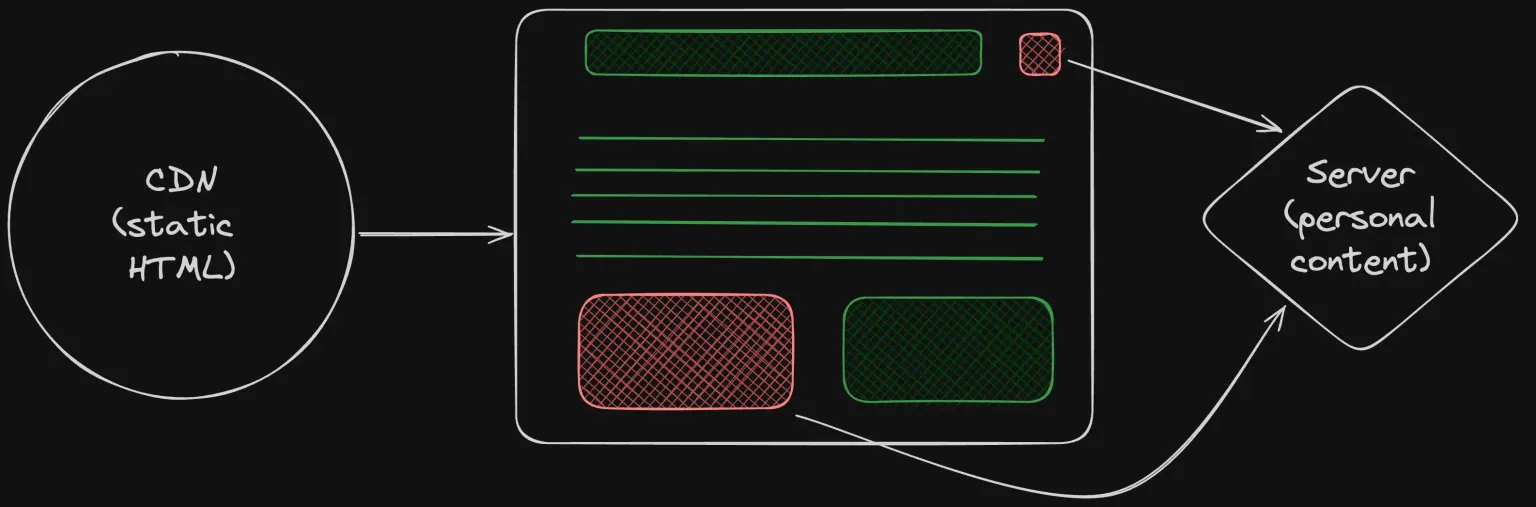
As shown, you can embed server-rendered content into a static page. The static parts can be cached by a CDN, while the server-rendered parts are fetched lazily after initial page load, maintaining fast initial load speed while increasing the content’s flexibility. This approach achieves a level of performance that wasn’t possible with traditional SSR.
To enable Server Islands, you simply add the server:defer
directive to your Astro components. For example, suppose you have a component that fetches a user’s avatar on the server side:
---import { getUserAvatar } from "../sessions";const userSession = Astro.cookies.get("session");const avatarURL = await getUserAvatar(userSession);---
<img alt="User avatar" src={avatarURL} />
In the page that uses this component, you just add the server:defer
directive:
---import Avatar from "../components/Avatar.astro";---
<!-- Other content here -->
<Avatar server:defer />
And that’s it! The page is statically generated, while the Avatar
component is server-rendered on demand. In addition, you can also specify fallback content to display while the server island is being loaded, akin to React’s Suspense
:
---import Avatar from "../components/Avatar.astro";import GenericAvatar from "../components/GenericAvatar.astro";---
<Avatar server:defer> <GenericAvatar slot="fallback" /></Avatar>
If you’d like a deeper explanation of how Server Islands differ from Astro’s standard Prerendering or On-demand Rendering, check out my article (in Japanese). It compares Next.js’s rendering methods and Astro’s Server Islands, giving you a broader understanding of modern rendering techniques.
Check out the official docs to learn more about Server Islands.
Astro DB
In March 2024, Astro introduced the @astrojs/db
package for database management. At first release, it was intended that production environments would connect to a managed database service called Astro Studio. As of now, you can connect to any libSQL server. For more about Astro Studio, see Related Projects.
To use Astro DB, you first need to define your table schema in db/config.ts
file:
import { defineTable, column } from "astro:db";
const Author = defineTable({ columns: { id: column.number({ primaryKey: true }), name: column.text(), }});
const Comment = defineTable({ columns: { authorId: column.number({ references: () => Author.columns.id }), body: column.text(), }});
export default defineDb({ tables: { Author, Comment },});
Then, in db/seed.ts
, export a function that seeds your database:
import { db, Comment, Author } from "astro:db";
export default async function() { await db.insert(Author).values([ { id: 1, name: "Kasim" }, { id: 2, name: "Mina" }, ]);
await db.insert(Comment).values([ { authorId: 1, body: "Hope you like Astro DB!" }, { authorId: 2, body: "Enjoy!"}, ])}
When you spin up the development server, the database is automatically created and seeded.
Querying the database go through the Drizzle client. The db
client imported from astro:db
is automatically configured to connect to the correct database, so you can just start querying right away:
---import { db, Comment } from "astro:db";
const comments = await db.select().from(Comment);---
<h2>Comments</h2>
{ comments.map(({ author, body }) => ( <article> <p>Author: {author}</p> <p>{body}</p> </article> ))}
With Astro DB, you can combine Astro’s simplicity of setup with the powerful query capabilities of Drizzle to manage databases in your Astro projects. Although it seems to be in the early stages yet, Astro DB already offers more than enough functionality for smaller features requiring a database. Definitely consifer testing it out in your next project!
Check out the official docs for more details on Astro DB.
astro:env
Astro 5.0 introduced astro:env
, a new way for handling environment variables type-safely. With astro:env
, you can:
- Specify usage context (client or server) to clarify how/where variables can be used
- Mark variables as secrets so they won’t be exposed to clients or inlined into the server build
- Specify whether a variable is required or optional, and verify required variables are set at server startup
- Define variable types, removing the need for manual type conversions in your application
You define your environment variable schema in your Astro config file:
import { defineConfig } from "astro/config";
export default defineConfig({ env: { schema: { // ... } }});
Then use the envField
helper to define fields:
import { defineConfig, envField } from "astro/config";
export default defineConfig({ env: { schema: { API_URL: envField.string({ context: "client", access: "public", optional: true }), PORT: envField.number({ context: "server", access: "public", default: 4321 }), API_SECRET: envField.string({ context: "server", access: "secret" }), } }});
Here, we use options like context
, access
, and optional
to specify properties like the context in which the variable can be accessed, whether it’s a secret, and if it’s optional. The variable types are declared by helper functions like envField.string
or envField.number
.
You can then import and use these variables from astro:env/*
:
---import { API_URL } from "astro:env/client";import { PORT } from "astro:env/server";import { API_SECRET } from "astro:env/server";---
Although it looks like a small feature, environment variables are often a source of headaches in application development, so having a built-in, type-safe approach is quite helpful.
Check out the official docs for setting up type-safe environment variables.
Request Rewriting
Astro 4.13 introduced Request Rewriting, a feature that allows you to serve a different route without redirecting the client to a new page. This means the browser URL remains the same, while the server reroutes the request to a different path behind the scenes, returning the result as if it were the response to the original request. This feature is particularly useful for scenarios like where you want to display the same content at multiple paths without maintaining different source files.
You can use rewrites by calling the Astro.rewrite
function in your Astro components:
---return Astro.rewrite("/login")---
Or in a middleware file:
import type { APIContext } from "astro";
export function GET({ rewrite }: APIContext) { return rewrite("/login");}
For more details on request rewriting, check out the official docs:
Container API
The Container API, which was at Stage 2 on Astro’s Project Roadmap in 2023, has been implemented in Astro 4.9 as an experimental feature. This feature enables you to render Astro components independently from the application context, much like the various methods offered by react-dom/server
in React. If you’re interested in learning more about the background of the Container API, check out my last year’s article for further details.
To use the Container API, you first need to create a container (the environment in which the component will be rendered) and call methods like renderToString
or renderToResponse
:
---import { experimental_AstroContainer } from "astro/container";import Card from "../src/components/Card.astro";
const container = await experimental_AstroContainer.create();const result = await container.renderToString(Card);---
result
will contain the rendered HTML of the Card
component, which you can use for testing purposes, such as checking if it contains a specific expected string. For a reference on how to write tests with the Container API, check out the official example project demonstrating how to use the Container API with Vitest.
Besides renderToString
, there’s also a renderToResponse
method, and you can pass props or slot data via various options. For more details on the Container API, see the official docs.
At Astro Together, there was a demo by Matthew Phillips showing how to use the Container API to embed Astro components (with islands!) into a PHP project, which was quite impressive. Be sure to check it out, too!
Related Projects
Astro Studio
Astro Studio launched as Astro’s first managed service in early 2024, but sadly announced its shutdown in September. The post explains that Astro Studio aimed to:
- Provide all Astro developers with an affordable, high-performance SQL data storage solution that is easy to use and integrated seamlessly with Astro
- Build a sustainable business model to support Astro’s continued development and growth
Unfortunately, the second point was not as successful as hoped, leading to the decision to shut down Astro Studio.
As for the shutdown timeline, it’s as follows:
- Invites to Astro Studio will remain closed.
- Existing users will no longer be able to create new databases after October 1, 2024.
- Existing databases will no longer be accessible after March 1, 2025.
- Any remaining databases will be deleted on or soon after March 1, 2025.
If you’re using Astro Studio, be sure to plan your migration to another database service in advance.
Meanwhile, Astro DB will still be available for use in your Astro projects. In v4.15, Astro DB introduced support for connecting to any libSQL server, so you can self-host a libSQL server or use a managed service like Turso to continue using Astro DB.
Astro Storefront
Astro Storefront is an e-commerce site project demonstrating many of Astro’s newer features mentioned above, including Actions and astro:env
. It represents the current state of Astro’s capabilities and is a great reference for building your own Astro projects. The Storefront source code is publicly available on GitHub, and the same code is used in the production for powering the Astro Swag Shop, where you can buy Astro merchandise.
Astro started life as a static site generator, but in recent years it has actively expanded its server-side functionality, now capable of building sites with dynamic content like e-commerce sites without sacrificing UX. Astro Storefront exemplifies this trend, and in March 2024, an E-commerce guide was also published to help you build your own e-commerce site with Astro.
The README of the Astro Storefront project covers design decisions and more, so combined with the code itself, it’s a great way to learn practical Astro development techniques. If you’re planning a serious Astro project, definitely check it out!
Astro Ecosystem CI
Ecosystem CI is a mechanism for preserving the overall quality of a software ecosystem. It originated in the Vite community, led mainly by dominikg:
Vite’s ecosystem is huge, with new libraries and tools constantly popping up. The flip side is that any unexpected breaking change in Vite can have a massive ripple effect. Ecosystem CI helps minimize that risk by continuously checking major ecosystem packages whenever Vite changes, ensuring things don’t unexpectedly break—or if they do, that they can be fixed promptly in collaboration with the package maintainers.
Ecosystem CI has spread beyond Vite to other projects like:
- Vue: vuejs/ecosystem-ci
- Svelte: sveltejs/svelte-ecosystem-ci
- Nuxt: nuxt/ecosystem-ci
- Nx: nrwl/nx-ecosystem-ci
- Vitest: vitest-dev/vitest-ecosystem-ci
- Rspack: rspack-contrib/rspack-ecosystem-ci, rspack-contrib/rsbuild-ecosystem-ci
- Biome: biomejs/ecosystem-ci
- Oxc: oxc-project/oxlint-ecosystem-ci
And now Astro has joined this trend with its own Ecosystem CI implementation, available at withastro/astro-ecosystem-ci.
You can see the results of the CI checks at https://github.com/withastro/astro-ecosystem-ci/actions. Each workflow has jobs for packages in the ecosystem (e.g. Starlight) and they test building, running, etc., to ensure compatibility with the withastro/astro package.
Ecosystem CI is more or less aimed at package developers, so if you’re simply using Astro, it’s not something you need to worry about. That said, if a package in the ecosystem suddenly breaks, Ecosystem CI could offer some useful insights into what’s going on. Even if you’re not directoly involved in open-source development, understanding the Ecosystem CI concept can help you maintain stability across internally developed packages, such as those used in your company systems.
Starlight
Starlight is a documentation theme released in 2023, which has now passed its first anniversary. Its version at the start of 2024 was v0.15.2, and it’s now at v0.30.3, reflecting the steady pace of updates. For example, v0.21.0 introduced the <FileTree>
component for displaying directory trees and the <Steps>
component for showing sequences of steps, and v0.27.0
added support for server-side rendering.
Adoption of Starlight soared last year with well-known projects like Biome and Knip, and it continues to see more usage in 2024. Some examples include:
- Cloudflare Docs
- jscodeshift (a codemod toolkit by Meta)
- Style Dictionary (a design token build system by Amazon)
- vlt /vōlt/ (a new package manager by isaacs, etc.)
Starlight’s UI has multilingual support, making it great for documentation sites in any language. If you need to build a documentation site, definitely consider Starlight as a candidate.
Clack and Bombshell
In June 2024, Nate Moore, an Astro co-creator, announced he would be leaving the core team:
After 3 incredible years with Astro, I’m ready for my next challenge! Building up @astrodotbuild from nothing with this team has been one of the most rewarding experiences of my life. 🙏
Devtools companies—have a CLI that needs some love? Let’s chat, DMs open! I’d love to help.
— Nate Moore (@n_moore) June 4, 2024
He noted he would now be focusing on Clack, a framework for building interactive CLI tools. Then in October, he revealed Bombshell that will host his projects going forward, including Clack:
— bombshell (@bombshell_dev) October 24, 2024
Clack provides CLI components like text inputs, multi-selects, spinners, and more, letting you easily build interactive CLI apps. You can check out a demo at https://www.clack.cc/ for an idea of its capabilities:
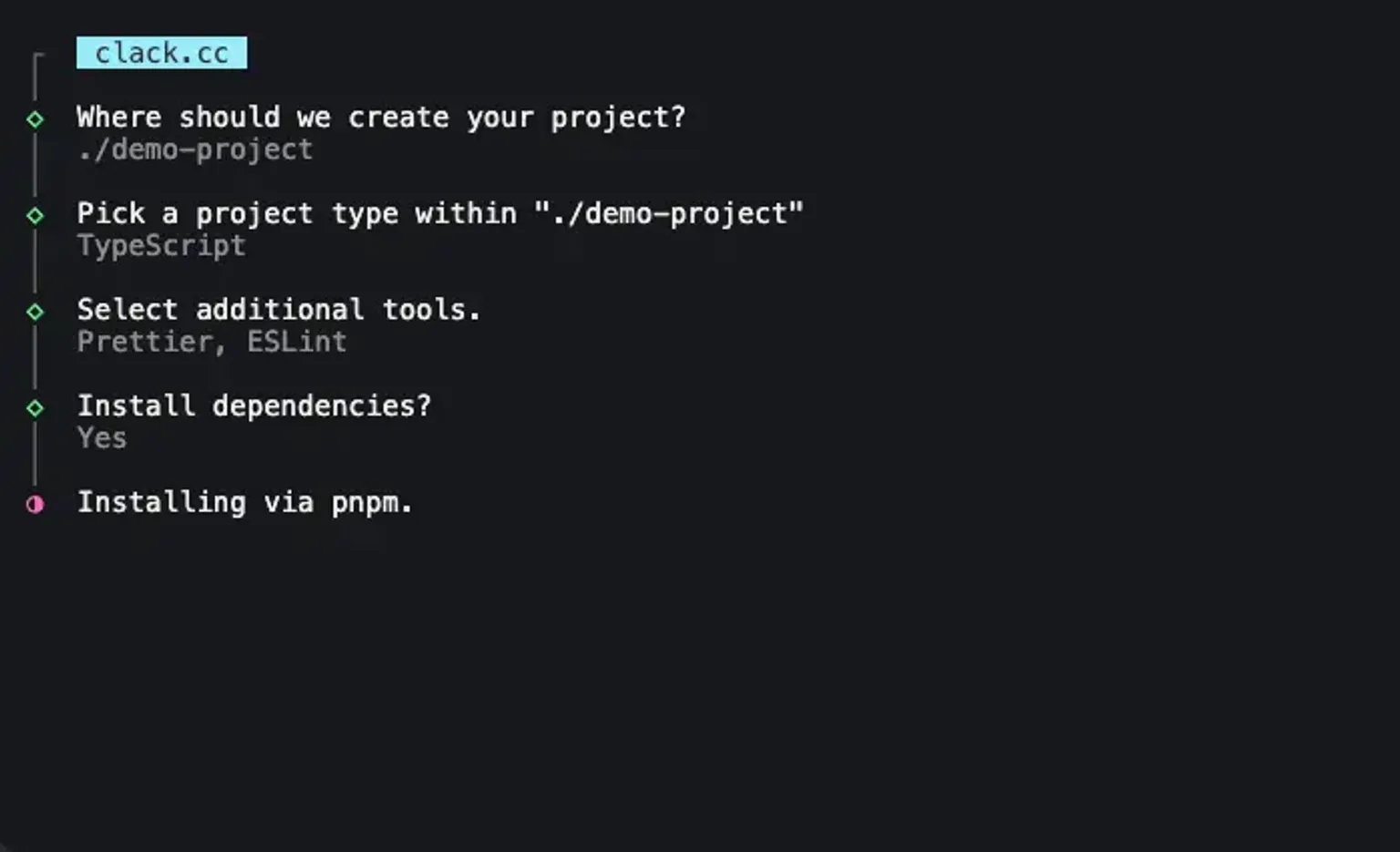
Docs are still under development, but Clack is already used by high-profile projects, including:
Find out more about Bombshell and Clack on the Bombshell website, and join their Discord server if you’d like to get involved.
Other Topics
Astro Together
On May 28, 2024, the first offline Astro meetup Astro Together was held in Motreal, Canada. There’s a blog post with a summary and photos of the event.
Talks from the event are available in a playlist on YouTube. The flow goes: Fred K. Schott on Astro’s current status and future, Ben Holmes demonstrating Astro Actions, Matthew Phillips explaining what changed in Astro 4.10, and Sarah Rainsberger talking about docs, Starlight, and the Astro community.
Several blog posts were also released around this event:
Bluesky
Astro now has its own Bluesky account. Bluesky provides a feature called Starter Packs, which bundles multiple related accounts under one theme so you can follow them all at once. Astro has its official Starter Pack, making it easy to keep track of Astro-related folks. Feel free to check it out!
Netlify as Official Deployment Partner
Netlify has become Astro’s official deployment partner. This means Netlify is now contributing $12,500 per month to support Astro’s development. As of that announcement, Netlify was also mentioned as helping with the development of Server Islands. Netlify is a popular choice for deploying Astro projects, so it’s great to see them supporting Astro’s development.
Netlify also uses Astro to run its developer platform site, which is another great example of Astro in action.
Google IDX as Official Online Editor Partner
Google IDX is now Astro’s official online editor partner.
Project IDX (Google IDX) is an online development environment similar to StackBlitz. Because it’s provided by Google, it can integrate with Gemini and Google Cloud. David East, who leads this project’s DevRel, has ties with Astro going back to his days on Firebase, including a project last year to rebuild the Firebase blog with Astro.
Through this partnership, Google IDX is sponsoring $10,000 each month to support Astro’s development.
Biome v1.6.0
Biome—a formatter and linter for JavaScript, JSON, CSS, and more—added partial support for Astro in its v1.6 release. v1.6 also introduced partial support for Vue and Svelte. For Astro, only the script portion inside code fences (---
) is supported so far. See Biome’s language support page for details.
Astro Storefront also uses Biome, so you can check that out for a real example.
Clerk Astro SDK
Clerk, a platform and components library for user authentication, released an SDK for Astro in July 2024.
I haven’t used it yet, but according to the announcement, it provides Astro components for sign-in, plus a clerkMiddleware
function for authentication checks in middleware, and more. Lucia is another well-known authentication library usable with Astro, but Clerk is more like an end-to-end solution. Having multiple authentication options is nice for the ecosystem.
See Clerk’s Astro SDK docs if you’re interested in adding authentication and authorization into your Astro project.
TutorialKit
TutorialKit is a tutorial-building tool developed by StackBlitz that uses Astro under the hood, leveraging WebContainer API. It lets you create fully interactive tutorials that run entirely in the browser. Check out the TutorialKit demo to see what it can do.
You can learn more about TutorialKit in their official docs. It’s a great tool for creating tutorials, so if you’re planning to write one, definitely give it a try!
IKEA, Porsche
Astro is now used by many websites, including big names like IKEA and Porsche:
IKEA’s site makes effective use of View Transitions, making navigation quite fun. Meanwhile, Porsche’s site runs Vue on Astro, making good use of Islands Architecture.
These are just two of many examples, and I’m sure there are many more fantastic Astro sites I’m not aware of. If you know of any other notable Astro sites, please share them with the Astro community by adding them to Companies & Websites Using Astro!
Looking Ahead to 2025
Astro has a public Project Roadmap where future development plans are discussed. The roadmap tracks proposals in four stages:
So if you want to see what’s coming up in Astro, check out what’s in Stage 2 and 3.
You can see the specific proposals under the “Public Roadmap” project. At the time of writing, the following five proposals are in Stage 3:
- Container API: render components in isolation
- Built-in SVG components
- Fonts
- Responsive images
- Sessions
Let’s take a look at some of these proposals below.
Sessions
Sessions are a mechanism to temporarily store user-specific data, such as login state or shopping carts. Up until now, you had to handle cookies or local storage directly. The new proposal would allow you to handle sessions natively in Astro. Data is stored server-side, and only the session ID is sent to the client as a cookie, which should help with security.
Sessions are already introduced experimentally in Astro 5.1. To work with sessions, you’ll use the Astro.session
object’s get
and set
methods. Sessions are available in many contexts, including pages, components, endpoints, actions, or middleware. Here’s an example from the article that demonstrates how to retrieve and update cart data in a session:
---export const prerender = false;const cart = await Astro.session.get("cart");---
<a href="/cart">🛒 {cart?.length ?? 0} items</a>
import { defineAction } from "astro:actions";import { z } from "astro:schema";
export const server = { addToCart: defineAction({ input: z.object({ productId: z.string() }), handler: async (input, context) => { const cart = await context.session.get("cart"); cart.push(input.productId); await context.session.set("cart", cart); return cart; }, }),};
Because sessions are still experimental, you must explicitly specify where to store that data using the driver
setting. For instance, here’s how to specify the file system driver when deploying to a Node.js environment:
{ adapter: node({ mode: "standalone" }), experimental: { session: { driver: "fs", }, },}
Which driver is available depends on your production environment. For example, you can also use drivers for Cloudflare KV or Redis. See the unstorage docs (which Astro Sessions uses internally) for a full list of drivers.
When sessions move out of experimental status, the plan is for the adapters themselves to pick the right driver automatically, so you won’t need to configure this manually in the future. If you spot any issues or have suggestions for improvements after trying it out, be sure to share your feedback at the PR of the proposal.
Responsive Images
Although images are a crucial factor in shaping user experience and therefore indispensable in web development, handling them can be quite challenging. Developers have to consider things like screen size, resolution, and image formats, etc. to ensure images are displayed correctly on various devices. The existing <Image>
component does offer a range of options to deal with these complexities, but it still requires a fair amount of effort on the developer’s part.
This proposal aims to introduce a layout
property that would automate the generation of srcset
and sizes
, letting developers easily follow image best practices.
It’s already available as an experimental feature in Astro 5.0, and you can check out the experimental responsive image docs for more details.
Fonts
Fonts are fundamental to web design, but Astro doesn’t currently provide a built-in approach for handling them. The official fonts guide can help you get started, but it still leaves lots of decisions like where to host fonts, how to load them, etc., up to the developer. This proposal aims to incorporate font best practices into Astro, making it easier to work with fonts in your projects.
Implementation details are still being discussed, but you can find the Astro Fonts RFC with examples of how configuration might look.
Closing Thoughts
That wraps up my personal overview of the new features and events that took place in the Astro ecosystem in this year. In 2024, we saw more application-focused enhancements, like Actions and Server Islands, though it’s unclear whether this direction will continue. The upcoming experimental features around images, fonts, and icons may indicate a further committment to the developer experience of essential, but often difficult tasks for managing content-focused sites. I’m looking forward to seeing what they come up with next.
I hope this article has inspired you to explore new Astro features and try experimenting with what’s possible. Thank you so much for reading this far!